JavaScript functions to get numbers in Indian format.
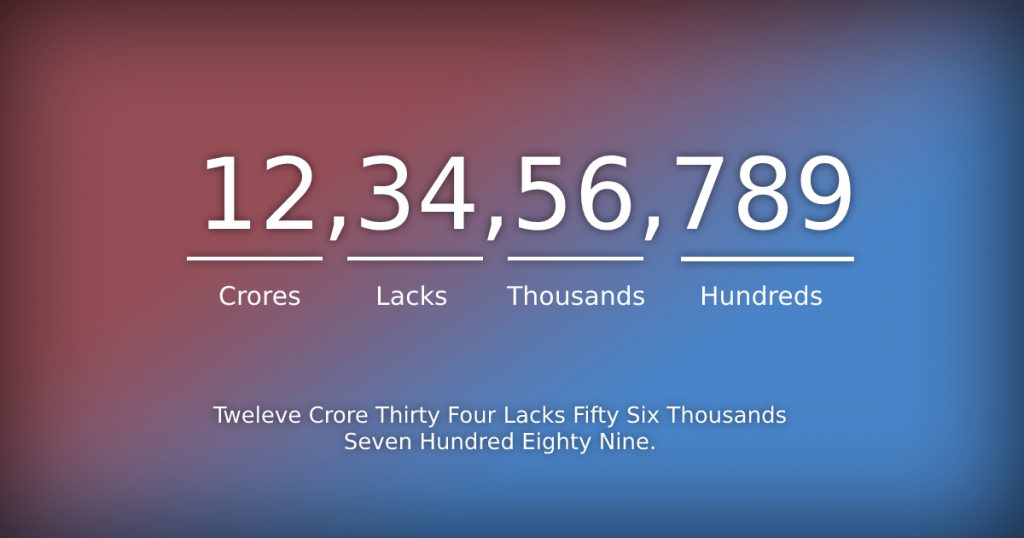
Doing things in javascript seems fun, there are loads of libraries available to achieve complex UI designs, but still few things are always left. one of them is converting a number into Indian format, not only into a comma-separated number but also in words.
Yes here comes the tricky part. having commas at places seems easy if you are an expert with string functions, but the word, wait a minute!! we follow the Crores and Lacks system in India, not the million or billions we used to hear in startup funding news.
Javascript makes things easy for us, just use the tools properly, and voila it will give us the great Indian format of any number. No need to say use this wisely, I mean make more sense when playing with currency numbers on your next website.
here it goes!!
To convert any integer number into a comma-separated Indian format number.
//get number in Indian format:
function indian_format(num)
{
var x=num;
x=x.toString();
var lastThree = x.substring(x.length-3);
var otherNumbers = x.substring(0,x.length-3);
if(otherNumbers != '')
lastThree = ',' + lastThree;
var res = otherNumbers.replace(/\B(?=(\d{2})+(?!\d))/g, ",") + lastThree;
return res;
}
To convert any integer number into words in Indian Format.
//get numbers in indian word format
function inWords(num) {
var a = ['','one ','two ','three ','four ', 'five ','six ','seven ','eight ','nine ','ten ','eleven ','twelve ','thirteen ','fourteen ','fifteen ','sixteen ','seventeen ','eighteen ','nineteen '];
var b = ['', '', 'twenty','thirty','forty','fifty', 'sixty','seventy','eighty','ninety'];
if ((num = num.toString()).length > 9) return 'out of limit';
n = ('000000000' + num).substr(-9).match(/^(\d{2})(\d{2})(\d{2})(\d{1})(\d{2})$/);
if (!n) return; var str = '';
str += (n[1] != 0) ? (a[Number(n[1])] || b[n[1][0]] + ' ' + a[n[1][1]]) + 'crore ' : '';
str += (n[2] != 0) ? (a[Number(n[2])] || b[n[2][0]] + ' ' + a[n[2][1]]) + 'lakh ' : '';
str += (n[3] != 0) ? (a[Number(n[3])] || b[n[3][0]] + ' ' + a[n[3][1]]) + 'thousand ' : '';
str += (n[4] != 0) ? (a[Number(n[4])] || b[n[4][0]] + ' ' + a[n[4][1]]) + 'hundred ' : '';
str += (n[5] != 0) ? ((str != '') ? 'and ' : '') + (a[Number(n[5])] || b[n[5][0]] + ' ' + a[n[5][1]]) + 'Rupees ' : '';
return str.charAt(0).toUpperCase() + str.slice(1);
}